Your Code Stinks If It Has These Code Smells
Code hygiene is one of most underrated activity in SDLC which silently hurts developer productivity, increases code complexity and bugs in code. One of the ways to keep code hygiene is to develop an eye for spotting code smells. Code smells are snippets which show improper usage of programming constructs. In this article, I am going to share some common types of code smells in js along with examples.
For those of you who are not satisfied with my simplified definition of code smells, here's the definition available on wikipedia -
Smells are certain structures in the code that indicate violation of fundamental design principles and negatively impact design quality.
Lets looks at some code smells commonly seen in javascript code -
XXL functions
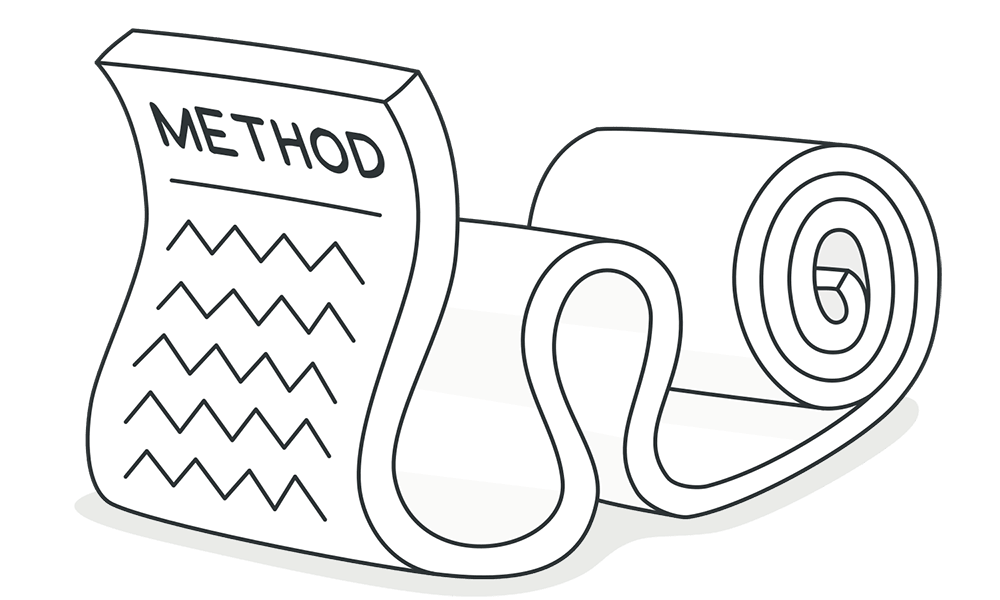
Extra extra large functions are quite common in react/node or any other web JS projects. Functions tend grow over time when they start handling multiple things in same place. I have seen functions as long as 300+ lines in codebase I have worked on. Not only XXL functions are really hard to understand but they also are extremely hard to test. XXL functions also are really difficult to debug. Overtime XXL functions become extremely difficult to maintain and lead to shotgun surgery.
Before
const justCookLunch = () => {
// order groceries { 20 lines }
// wash & cut vegetables { 10 lines }
// cook curry { 50 lines }
};
After
const justCookLunch = () => {
// order groceries { 20 lines }
// wash & cut vegetables { 10 lines }
// cook curry { 50 lines }
};
const orderGroceries = (store, groceryList) => {
// order groceries from store
};
const washVegetables = (vegetablesList) => {
// wash vegetables
};
const cutVegetables = (vegetablesList) => {
// cut vegetables
};
const cookDish = (dishName) => {
// cook curry
};
Every function should do one thing and one thing ONLY. Ideally a function should not grow larger than 20 lines of code. Few of the other code smells related to functions include:
- more than 5 function parameters
- really long names or difficult to understand names
Duplicate Code
DRY ( Don't Repeat Yourself ) is one of the most under-utilized principles in programming. Duplicate code is often an after-effect of violating DRY. Either a lack of understanding of the existing utility functions or laziness is what leads to duplicate piece of code lying around at multiple places across the codebase. Duplicate code increases maintenance cost and also makes the codebase error prone. Every time you change the code at one place, you are forced to make the changes across all the locations where the code is duplicated.
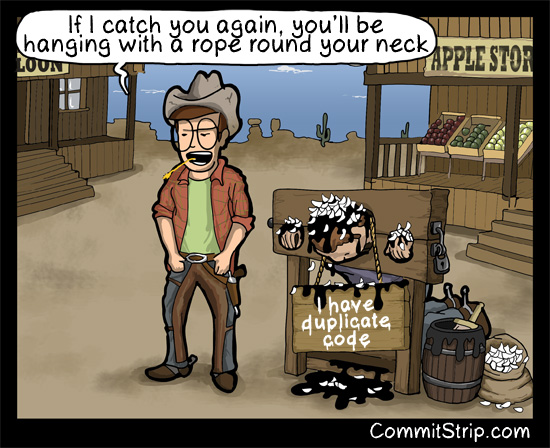
If you ever spot a duplicate code, follow the boy scout rule and create a util function which can be reused.
Dead Code
Dead code is the often a low hanging fruit which goes unnoticed. Unused functions, unused enums, commented code, deprecated modules, unused imports all are examples of dead code. There are tons of tools and libraries which you can use to highlight and fix dead code. Cleaning up dead code often will help you increase your code coverage.
If you are building react based web apps, you can use webpack-deadcode-plugin webpack plugin to detect unused files and exports in your projects.
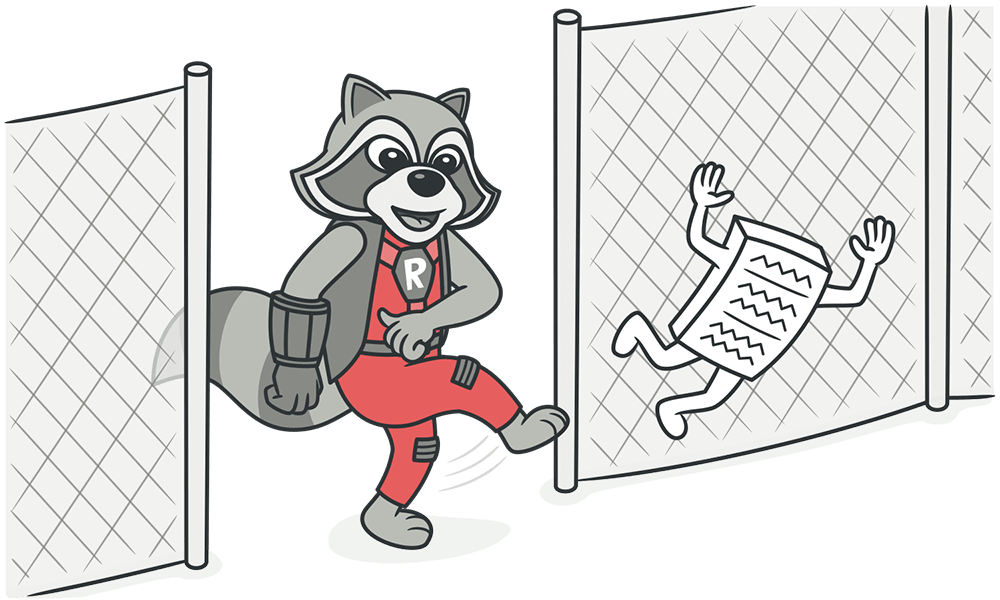