Notes on RPC(Remote Procedure Call)
RPC stands for Remote Procedure Call. It's a communication paradigm== used in distributed systems to allow a program running on one computer to execute a procedure (function) on a different computer like a local procedure call.
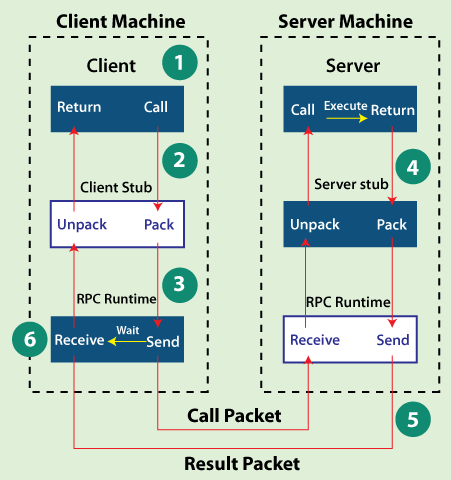
Key components of RPC
-
Client: The client is the program that initiates the RPC call. It's the one that knows what procedure to call and what arguments to pass.
-
Client Stub: The client stub is a piece of code that sits on the client side. It's responsible for marshalling the arguments, sending the request over the network, and receiving the response.
Client stubs can use various formats for marshalling the arguments. This includes formats like JSON, XML, or binary.
-
Server: The server is the program that provides the service being called. It's the one that actually executes the procedure.
-
Server Stub: The server stub is a piece of code that sits on the server side. It's responsible for receiving the request, unmarshalling the arguments, executing the procedure, and sending the response back to the client.
-
Network Protocol: The network protocol is the set of rules and conventions that govern how the client and server communicate with each other. This can include protocols like TCP/IP, HTTP, or custom protocols.
-
IDL (Interface Definition Language): IDL is a language used to define the interface between the client and server. It specifies the procedures that can be called, the arguments they take, and the return values.
Here's a quick illustration of how these components interact:
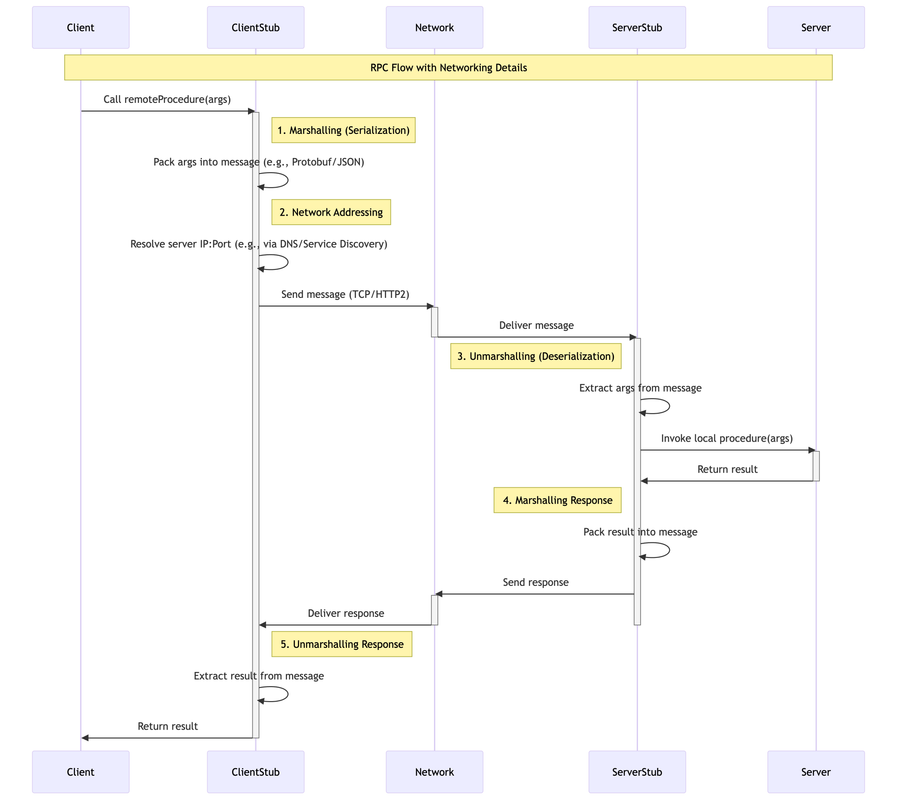
Why do we need RPC, when we already had HTTP (or REST) ?
-
REST over HTTP carries extra metadata (has headers, uses JSON/XML request/responses ) in nature requiring higher network bandwidth and thereby increasing latency.
-
HTTP doesnt support retries, timeouts etc. these have to be built by developers. RPC frameworks like gRPC provide these features out of the box.
-
RPC is language and platform agnostic. The client and server stubs are generated from the IDL.
Implmenting simple RPC with gRPC
gRPC is a framework developed by Google. gRPC uses Protocol Buffers (protobuf) as its interface definition language (IDL) and HTTP/2 as its transport protocol.
Lets implment a simple RPC service using gRPC step by step in js.
- Define the Service Definition
Create a file named hello.proto in your project directory:
- Generate gRPC JavaScript Code
This command uses the grpc_tools_node_protoc tool to generate two JavaScript files:
- hello_pb.js: Contains the JavaScript code for the HelloRequest and HelloReply message types.
- hello_grpc_pb.js: Contains the JavaScript code for the Greeter service definition and the client/server stubs.
- Implement the gRPC Server (hello_server.js):
Create a file named hello_server.js:
In this code:
- We import the necessary grpc and proto-loader libraries.
- We define the path to our .proto file and load its definition using protoLoader.loadSync.
- We load the helloworld package from the loaded definition.
- The sayHello function implements the logic for the SayHello RPC. It takes the call object (containing the request) and a callback function. We call the callback with null for the error and a response object containing the greeting.
- The main function creates a new gRPC server, adds our Greeter service implementation, binds it to port 50051 using insecure credentials (for simplicity in this example), and starts the server.
- Implement the gRPC Client (hello_client.js)
Create a file named hello_client.js
In this code:
- We again import the necessary libraries and load the .proto definition.
- In the main function, we create a gRPC client by instantiating hello_proto.Greeter with the server address (localhost:50051) and insecure credentials.
- We get the name to greet from the command line arguments or default to "World".
- We create a HelloRequest object with the provided name.
- We call the sayHello method on the client stub, passing the request and a callback function. The callback handles the response (or any error) from the server and logs the greeting.
- Run the Server and Client
- Run the server: node hello_server.js
- Run the client: node hello_client.js Nathan
This example demonstrates a basic unary RPC in JavaScript using gRPC. The client sends a single request (HelloRequest) to the server, and the server sends back a single response (HelloReply). gRPC handles the underlying serialization (using Protocol Buffers), transport (using HTTP/2), and deserialization, allowing you to focus on defining your service and implementing the business logic.
RPC vs REST
Criteria | RPC (Remote Procedure Call) | REST (Representational State Transfer) |
---|---|---|
Definition | Protocol that allows executing procedures on remote systems | Architectural style for networked applications using HTTP |
Communication Style | Action-oriented (calls methods/functions) | Resource-oriented (manipulates representations of resources) |
Protocol | Can use various protocols (gRPC, JSON-RPC, XML-RPC) | Typically uses HTTP/HTTPS |
Data Format | Depends on implementation (Protocol Buffers, JSON, XML) | Typically JSON or XML |
Performance | Generally faster due to binary protocols (e.g., gRPC) | Slightly slower due to text-based formats |
When to Use |
|
|
When Not to Use |
|
|
Companies Using |
|
|
Use Cases |
|
|