Beginner's guide to DOM manipulation
DOM stands for Document Object Model. Every html page is internally(to browser) represented as a tree of objects. This tree is called the DOM tree.
Whenever you want to change the content of the page dynmically, e.g if a user clicks a button and you would like to show a message on the page, you need to use DOM manipulation.
To manipulate the DOM you need to be able to access it. To do that, you need to use the document object.
Document object is a global object in the browser. It is the root of the DOM tree. It represents the html page. It has properties and methods that allow you to access and manipulate the html page.
Here's how you can access the document object in the browser console.
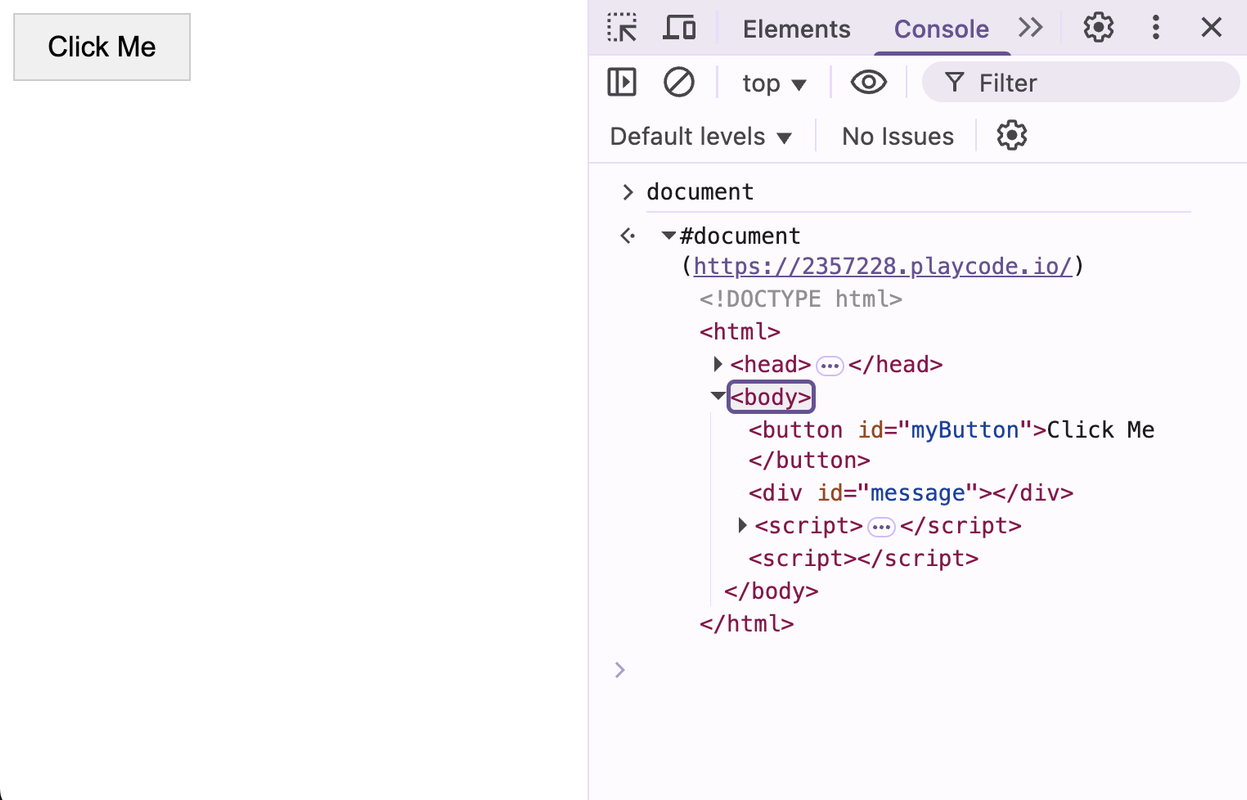
For the scope of this article, we are going to use this hosted html page - https://2357228.playcode.io/
The code for this page is as follows.
Accessings the DOM tree
We will use the methods of document object to access the DOM tree. Here's a list of methods which enable us to access the DOM tree.
- getElementById()
This method returns the element with the specified id. This method returns a single element in HTMLElement type object.
- getElementsByClassName()
This method returns the elements with the specified class name. This method returns a list of elements in HTMLCollection type object.
- getElementsByTagName()
This method returns the elements with the specified tag name. This method returns a list of elements in HTMLCollection type object.
- querySelector()
This method returns the first element that matches the specified CSS selector. This method returns a single element in HTMLElement type object.
- querySelectorAll()
This method returns all the elements that match the specified CSS selector. This method returns a list of elements in NodeList type object.
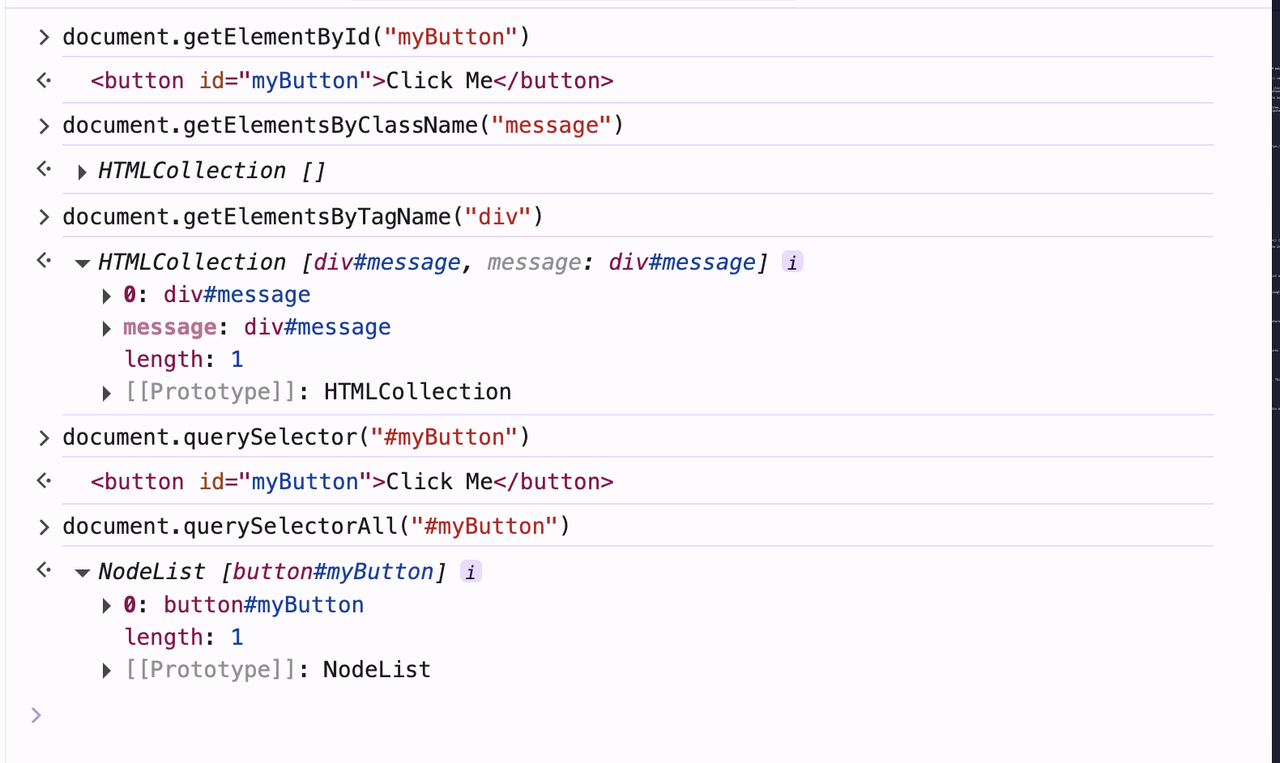
Manipulating the DOM tree
Once you have access to the DOM tree, you can manipulate it. You can add remove elements from tree, you can change the content of the elements, you can even modify the properties of the elements.
Let's see how we can do this.
Modifying the content of the elements
- element.textContent
You can modify the content of the element using the textContent property. First you need to access the element using the methods we discussed above. Then you can use the textContent property to modify the content of the element.
- element.innerHTML
You can modify the content of the element using the innerHTML property. First you need to access the element using the methods we discussed above. Then you can use the innerHTML property to modify the content of the element.
Try out sample modifications below in the browser console.
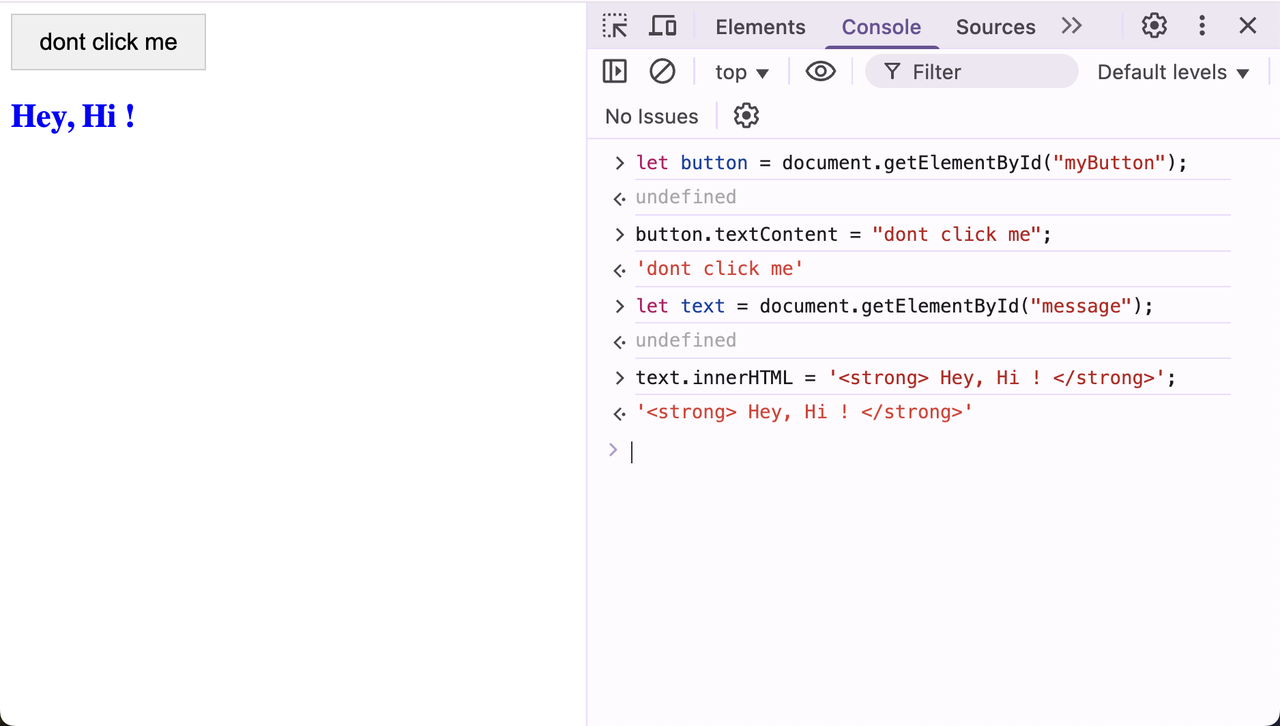
Modifying the properties of the elements
-
element.setAttribute() - You can modify the properties of the element using the setAttribute() method.
-
element.removeAttribute() - You can remove the properties of the element using the removeAttribute() method.
Adding and removing elements from the DOM tree
-
element.appendChild() - You can add an element to the DOM tree using the appendChild() method.
-
element.removeChild() - You can remove an element from the DOM tree using the removeChild() method.
-
element.replaceChild() - You can replace an element from the DOM tree using the replaceChild() method.
-
element.cloneNode() - You can clone an element from the DOM tree using the cloneNode() method.
-
element.insertBefore() - You can insert an element before another element in the DOM tree using the insertBefore() method.
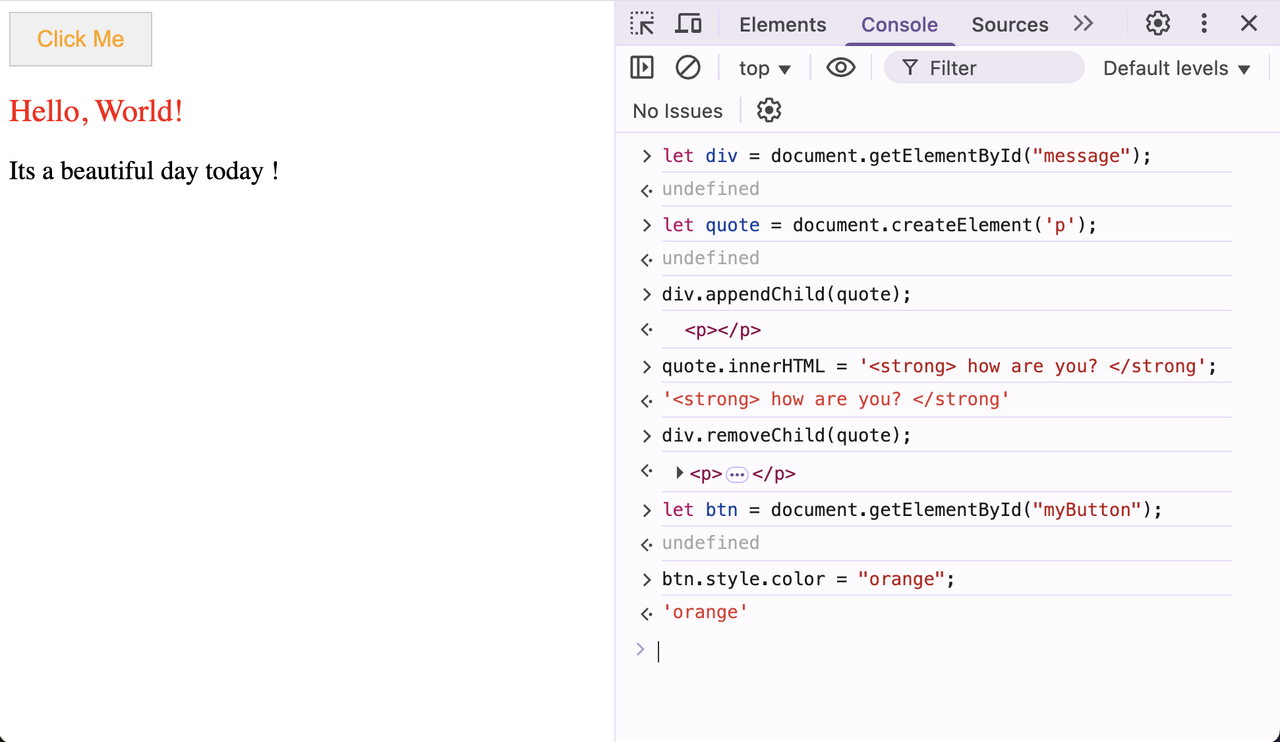
Event handling
To make your website interactive, you need to handle events. Events are actions that happen in the browser, such as a user clicking a button, hovering over an element, or submitting a form. To be more specific -
- Mouse events: click, mouseover, mouseout, mousedown, mouseup, mousemove
- Keyboard events: keydown, keyup, keypress
- Form events: submit, change, focus, blur
- Document/Window events: load, DOMContentLoaded, resize, scroll
You can add event listeners to the elements in the DOM tree. You can add event listeners to the elements using the addEventListener() method.
- type - The type of the event.
- listener - The function to be called when the event occurs.
- options - The options for the event listener.
When an event occurs, an event object is created and passed as an argument to the event listener function. This object contains information about the event, such as the target element, the type of event, and any specific data related to the event (e.g., mouse coordinates, key pressed)
Here's how you can add an event listener to the button element.
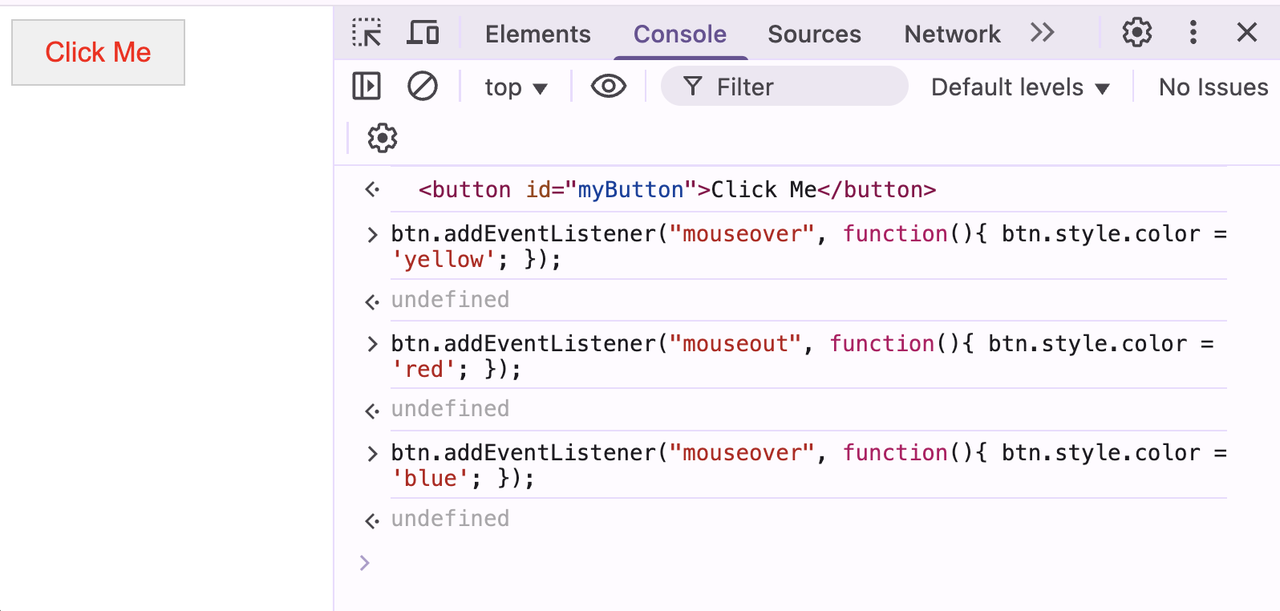
If you refer our original sample page, you would notice that we have click event listener on the button.
Event Propagation
An event goes through three phases:
-
Capturing phase - The event starts at the top of the DOM tree and trickles down to the target element.
-
Target phase - The event reaches the target element.
-
Bubbling phase - The event bubbles up from the target element to the top of the DOM tree. This is the default phase.
Event Delegation
Event delegation is a technique for adding event listeners to a parent element instead of adding them to each child element. This is useful when you have a lot of child elements and you don't want to add event listeners to each of them.
Here's a quick example -